Mach is still early stages - see what we have today and stay tuned.
The WebGPU interface for Zig
Mach core vs. engine
All examples below are Mach core examples: Mach provides truly-cross-platform window+input+WebGPU API, nothing else! These examples show how to leverage WebGPU in Zig for graphics.
These are not Mach engine examples (which would be higher-level): they're low-level, Mach just handles the cross-platform bits (providing you with browser, mobile, and more support in the future.)
mach/gpu
mach/gpu leverages Google Chrome's WebGPU implementation behind the scenes: we do some heavy lifting to give you effortless cross-compilation and make it all 'just work' with no hassle in under 60 seconds on Windows/Linux/macOS.
WebGPU is an up-and-coming low-level graphics API like Vulkan, Metal, and DirectX 12. In the future, Mach will provide higher-level _Mach engine_ examples with model rendering, text rendering, etc. as optional libraries on built on top. You get to pick & choose what you use!
advanced-gen-texture-light example
Generates a brick texture at comptime, uses Blinn-Phong lighting, and several pipelines. Move camera with arrow keys / WASD.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-advanced-gen-texture-light
textured-cube example
Loads a PNG image and uploads the texture to the GPU. Renders it on a 3D cube.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-textured-cube
cubemap example
Renders a cubemap / skybox. Nothing fancy, but these are instrumental as backgrounds in games.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-cubemap
boids example
Uses a GPU compute shader to run calculations / simulate flocking behaviour of birds.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-boids
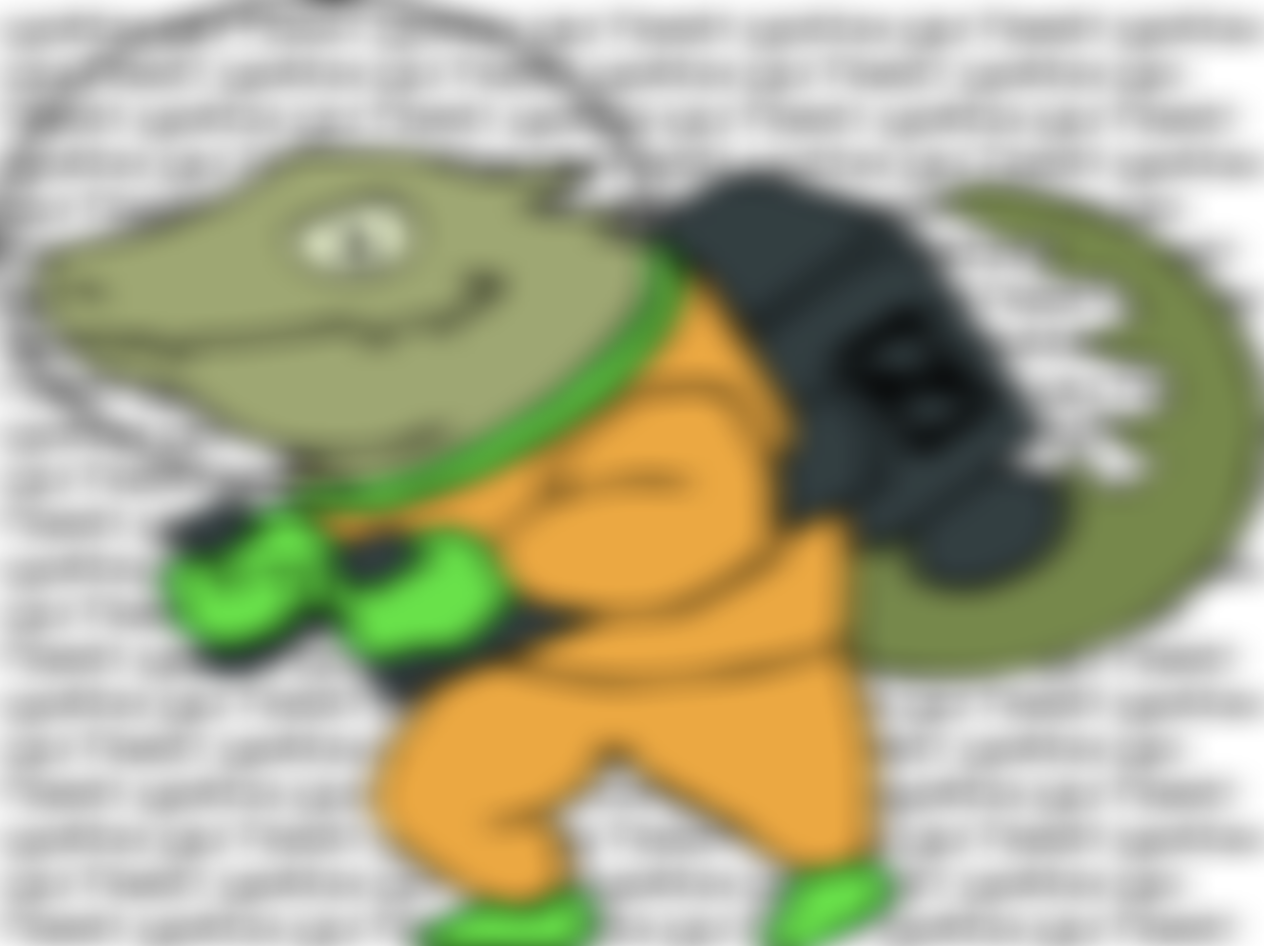
image-blur example
Leverages a compute shader to blur an image, then renders it. Don't worry if the details are a bit fuzzy!
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-image-blur
triangle example
This is where you should start learning. It tells the GPU to render 3 vertices (but doesn't transfer them using a vertex buffer or anything! The vertex positions are hard-coded in the shader.)
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-triangle
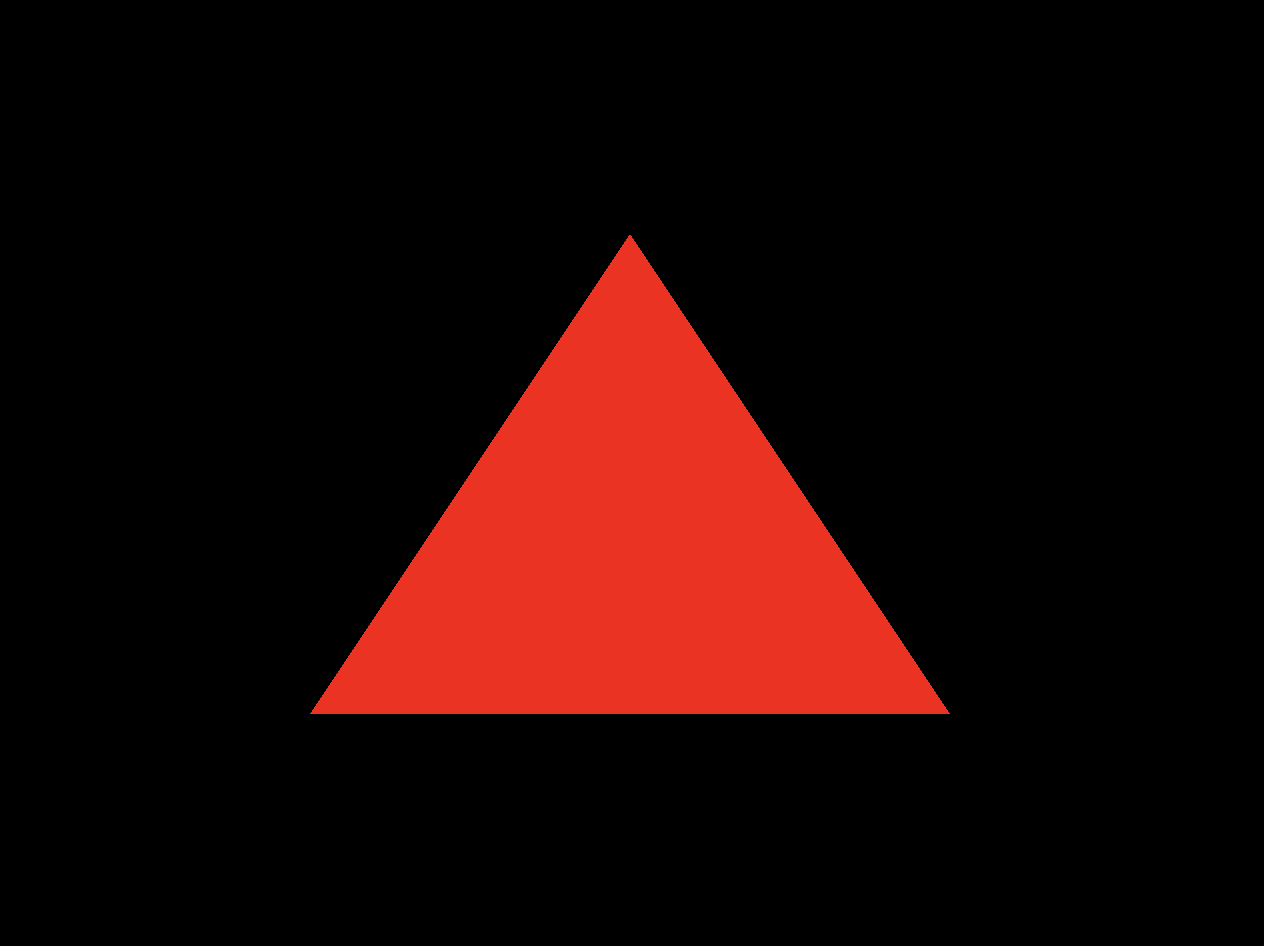
rotating-cube example
Uploads a basic 3D cube to the GPU and renders it. Demonstrates how to use vertex buffers to transfer a model from the CPU to GPU, how to use vertex attributes & more.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-rotating-cube
two-cubes example
Once you've learned how to render one cube, two is just 30 lines of code more!
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-two-cubes
instanced-cube example
EVEN MORE CUBES! Instancing lets you duplicate an object & render it in multiple places with different parameters.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-instanced-cube
fractal-cube example
Cube-inception! Renders the scene to a texture, which is then rendered on the rotating cube itself as a texture!
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-fractal-cube
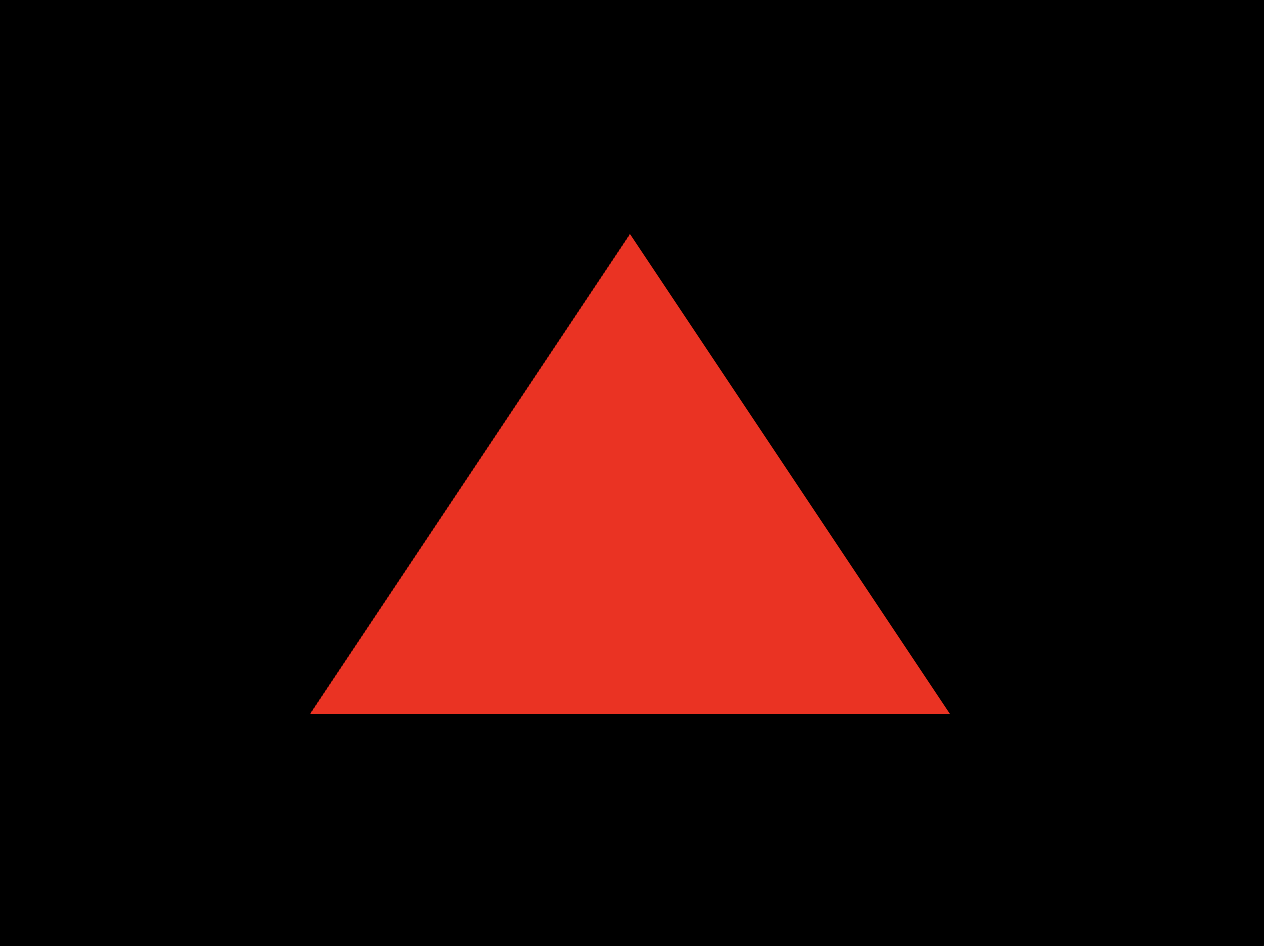
triangle-msaa example
Remember that triangle from before? If we turn on MSAA (Multi-Sample Anti Aliasing) the edges will become smooooth.
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-triangle-msaa
map-async example
Some of the best examples have no graphics. This one shows how to transfer data to the GPU, perform computations on that data using the GPU's parallel processing capbilities, and get results back on the CPU. If you're interested in GPU compute, this is the place to start!
git clone --recursive https://github.com/hexops/mach-examples
cd mach-examples/
zig build run-map-async
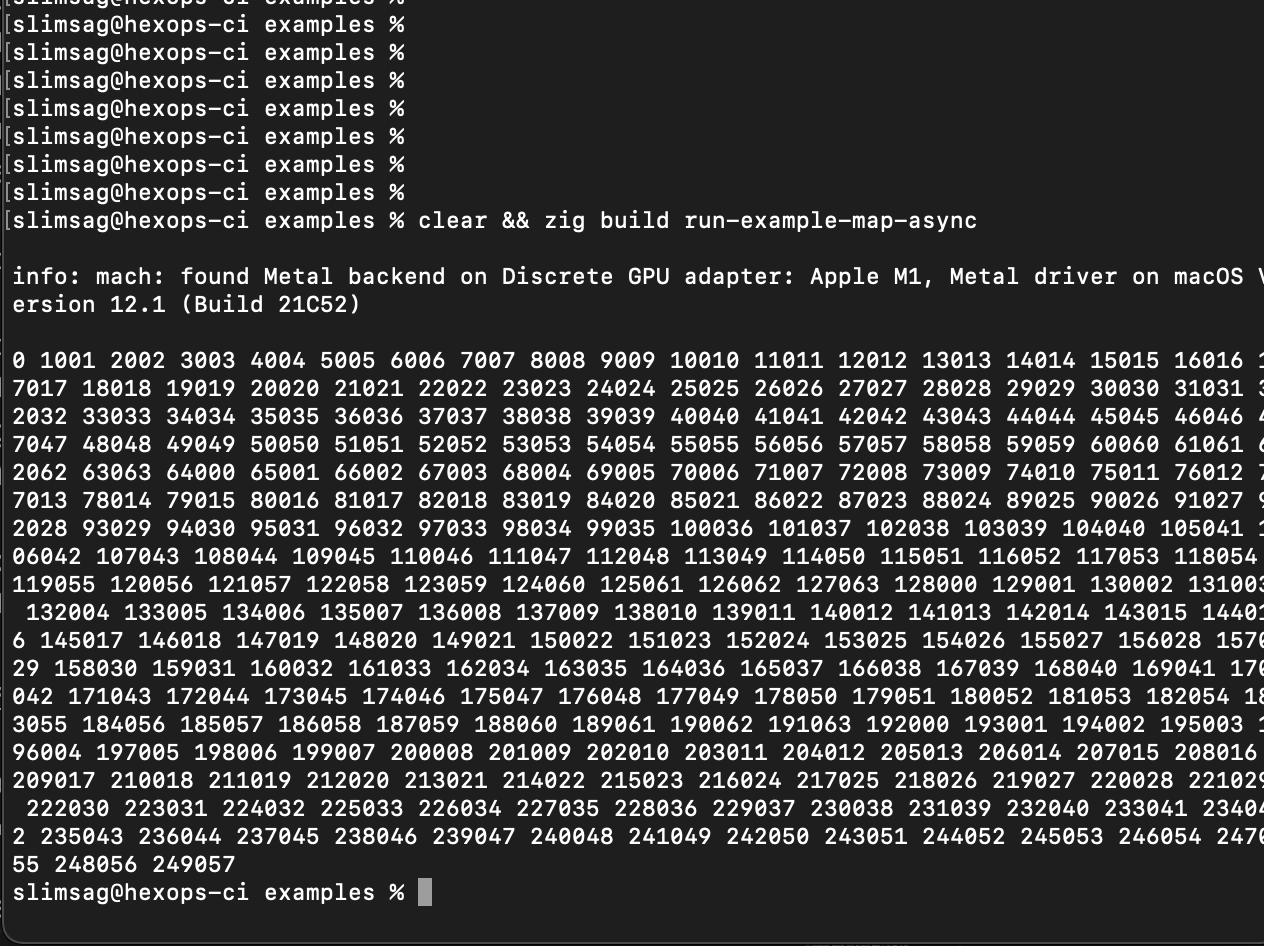
Join the community!
Join the Discord server - we'd love to have you there and are always happy to help!
We're also looking for more contributors to help us build and port WebGPU examples for Zig. If you prefer reading about WebGPU, there's plenty of great learning material online about compute and rendering[0][1].
Special thanks to these people who've contributed to these examples: