Mach core examples
All examples require this nominated Zig version | known issuesgen-texture-light
Generates a brick texture at comptime, uses Blinn-Phong lighting, and several pipelines. Move camera with arrow keys / WASD.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-gen-texture-light
procedural-primitives
Procedurally generated geometry, implements a few different shapes (use arrow keys to switch between them.)
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-procedural-primitives
pixel-post-process
A post-processing effect which pixelates the entire screen.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-pixel-post-process
sprite2d
Loads a JSON file which describes a spritesheet, and renders sprites to the screen.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-sprite2d
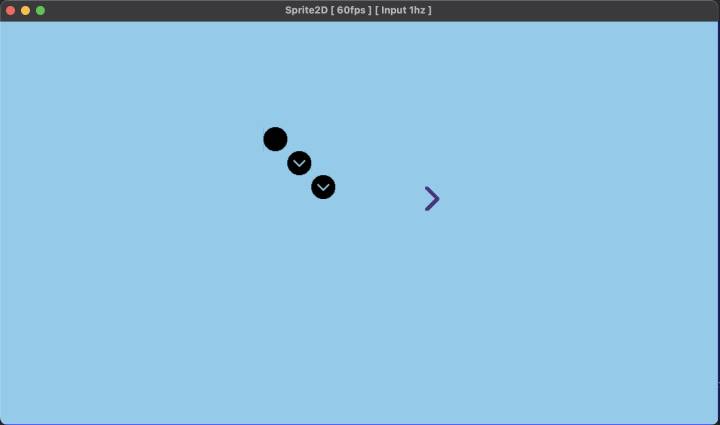
fractal-cube
Cube-inception! Renders the scene to a texture, which is then rendered on the rotating cube itself as a texture!
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-fractal-cube
cubemap
Renders a cubemap / skybox. Nothing fancy, but these are instrumental as backgrounds in games.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-cubemap
textured-cube
Loads a PNG image and uploads the texture to the GPU. Renders it on a 3D cube.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-textured-cube
image-blur
Leverages a compute shader to blur an image, then renders it. Don't worry if the details are a bit fuzzy!
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-image-blur
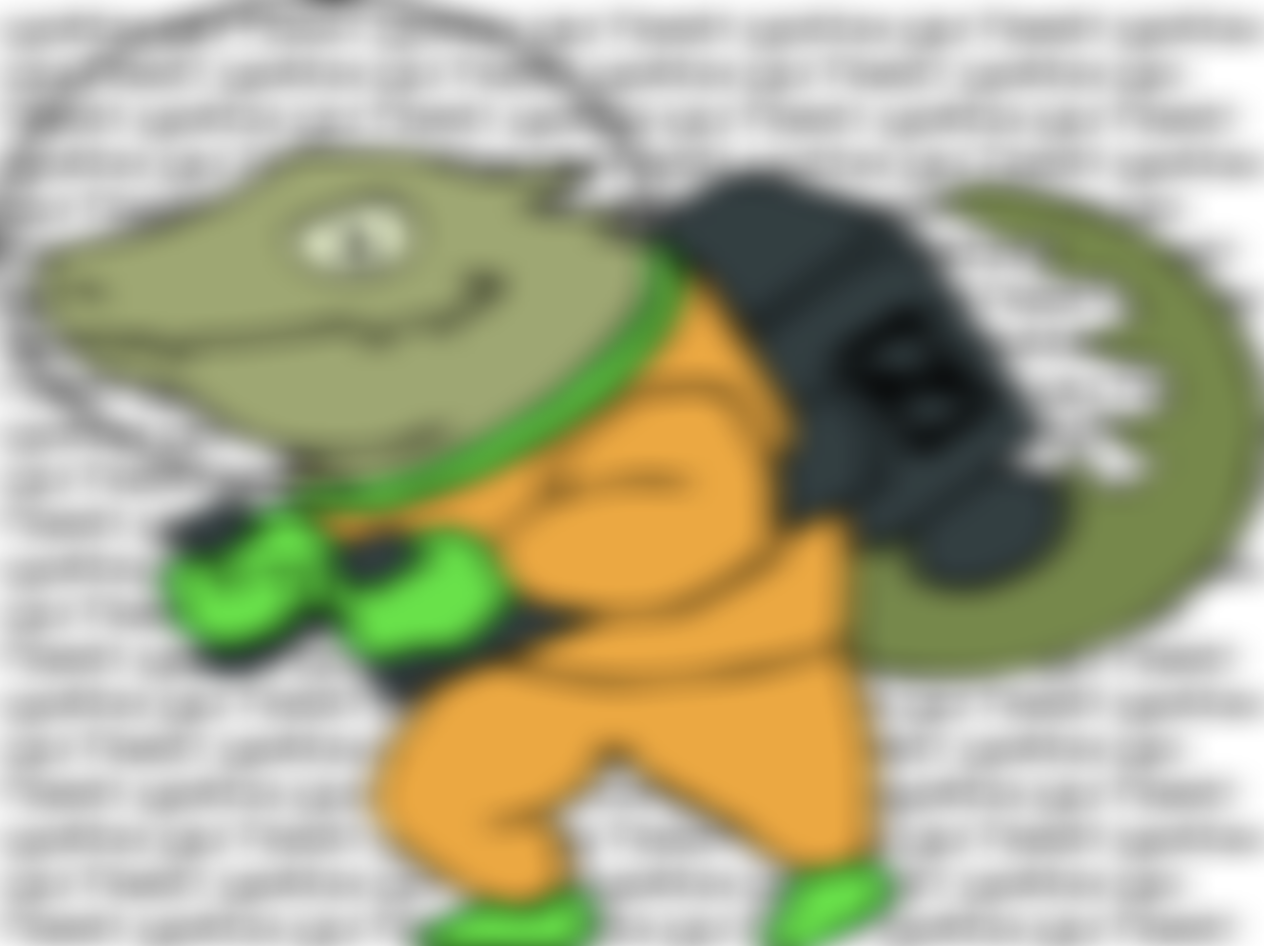
boids
Uses a GPU compute shader to run calculations / simulate flocking behaviour of birds.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-boids
two-cubes
Once you've learned how to render one cube, two is just 30 lines of code more!
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-two-cubes
instanced-cube
EVEN MORE CUBES! Instancing lets you duplicate an object & render it in multiple places with different parameters.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-instanced-cube
rotating-cube
Uploads a basic 3D cube to the GPU and renders it. Demonstrates how to use vertex buffers to transfer a model from the CPU to GPU, how to use vertex attributes & more.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-rotating-cube
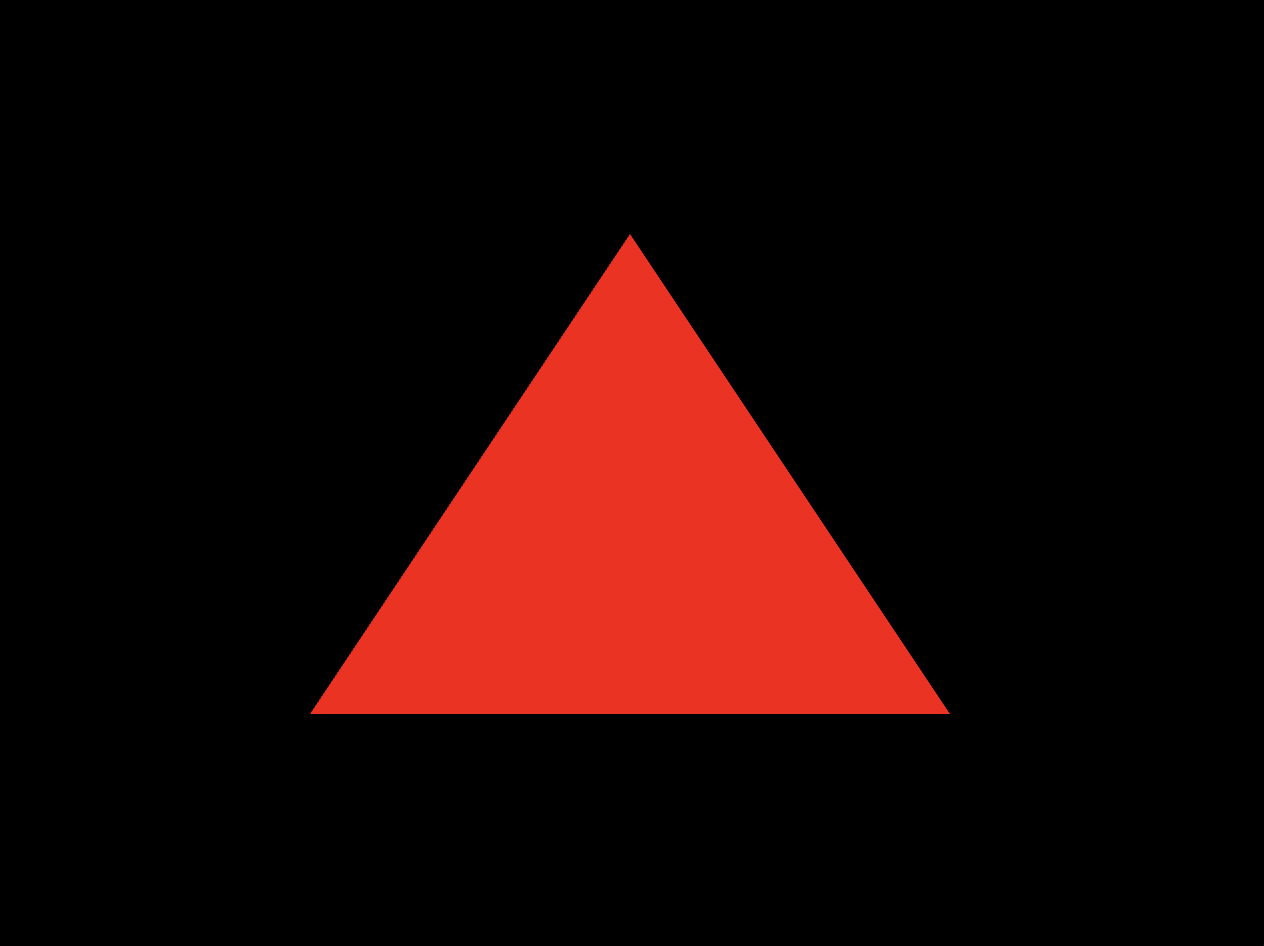
triangle-msaa
Remember that triangle from before? If we turn on MSAA (Multi-Sample Anti Aliasing) the edges will become smooooth.
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-triangle-msaa
triangle
This is where you should start learning. It tells the GPU to render 3 vertices (but doesn't transfer them using a vertex buffer or anything! The vertex positions are hard-coded in the shader.)
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-triangle
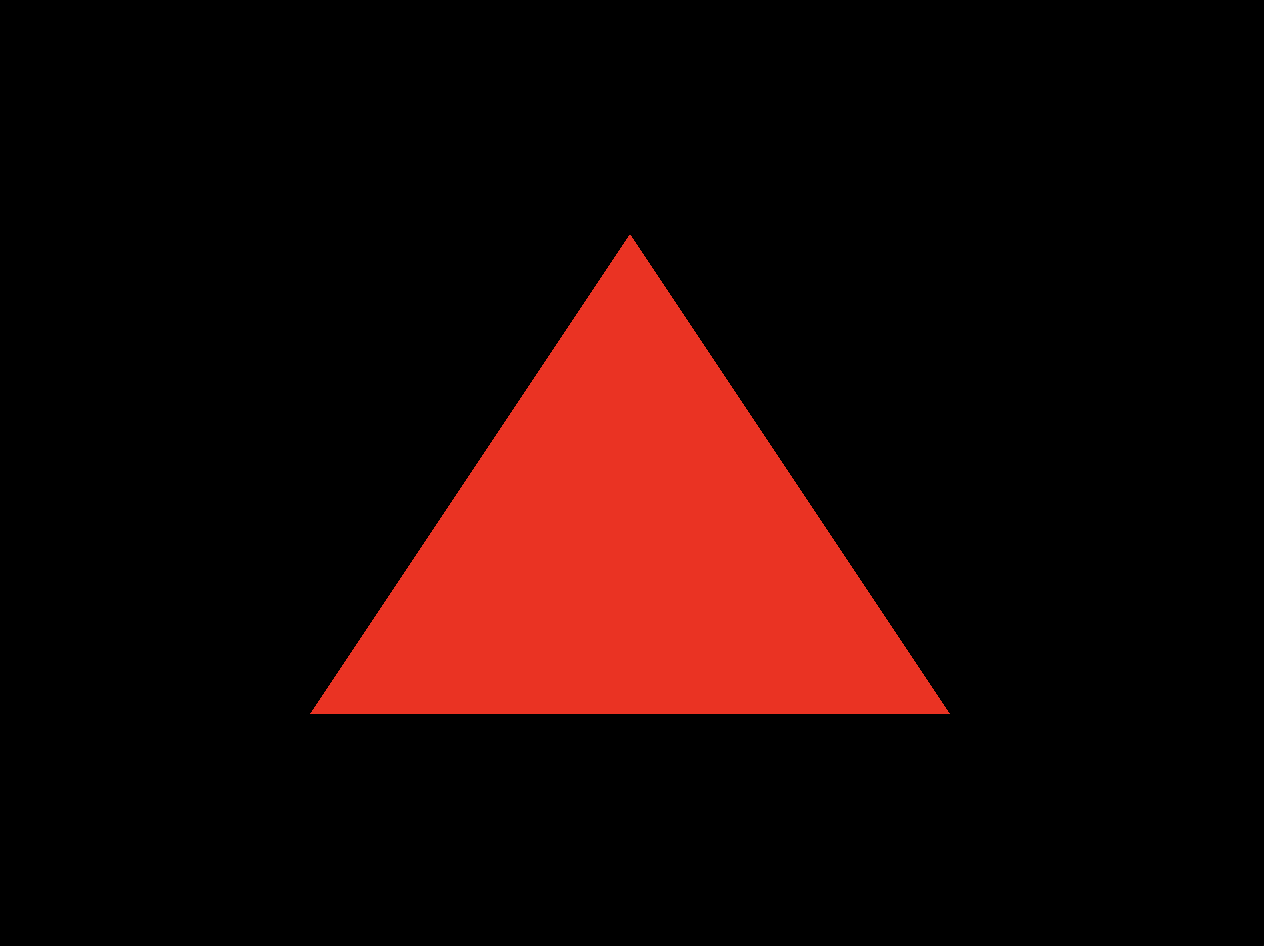
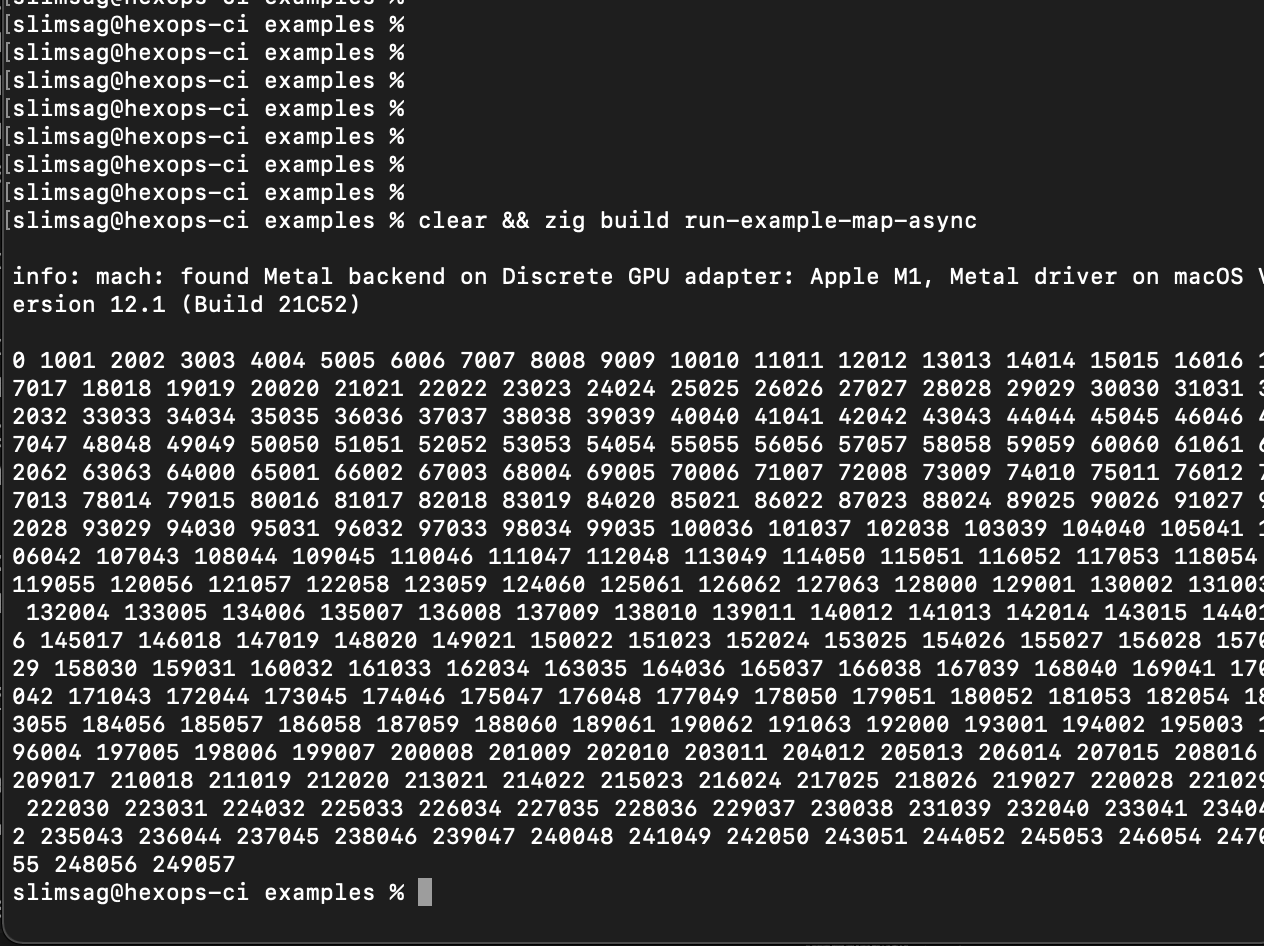
map-async
Some of the best examples have no graphics. This one shows how to transfer data to the GPU, perform computations on that data using the GPU's parallel processing capabilities, and get results back on the CPU. If you're interested in GPU compute, this is the place to start!
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-map-async
clear-color
As simple as it gets: a blue screen of doom
git clone https://github.com/hexops/mach
cd mach/
zig build run-core-clear-color
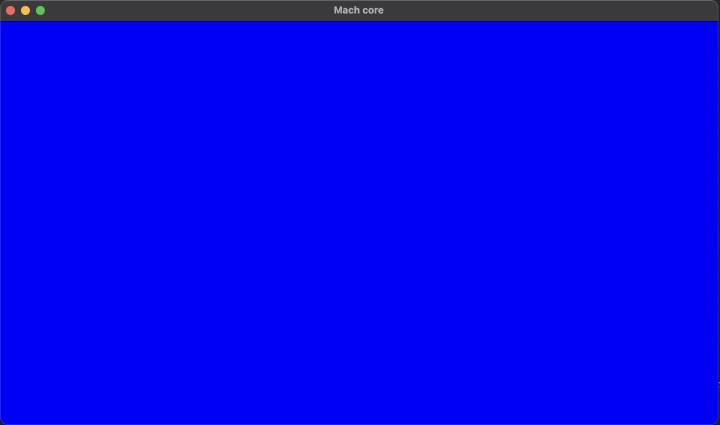
Learning
You can start with the simplest examples first. There’s also plenty of great learning material online for WebGPU (including video tutorials.)
Lines of code | Example |
---|---|
79 | clear-color |
80 | triangle |
86 | map-async |
123 | triangle-msaa |
221 | rotating-cube |
230 | instanced-cube |
243 | two-cubes |
294 | boids |
322 | image-blur |
337 | textured-cube |
378 | cubemap |
381 | fractal-cube |
421 | sprite2d |
547 | pixel-post-process |
588 | procedural-primitives |
780 | gen-texture-light |
Contribute
We're looking for more contributors to help us build and port a few more WebGPU examples to Zig. There's plenty of great learning material online for both rendering and compute.
Join our Discord server - we'd love to have you there and are happy to help!
Special thanks to these people who've contributed to these examples: